2011-02-07 07:48:44 +01:00
# Sitetree
## Introduction
2012-06-27 16:09:21 +02:00
Basic data-object representing all pages within the site tree.
The omnipresent *Page* class (located in `mysite/code/Page.php` ) is based on this class.
## Creating, Modifying and Finding Pages
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
See the ["datamodel" topic ](/topics/datamodel ).
2011-02-07 07:48:44 +01:00
## Linking
:::php
// wrong
$mylink = $mypage->URLSegment;
// right
$mylink = $mypage->Link(); // alternatively: AbsoluteLink(), RelativeLink()
2012-06-27 16:09:21 +02:00
In a nutshell, the nested URLs feature means that your site URLs now reflect the actual parent/child page structure of
your site. The URLs map directly to the chain of parent and child pages. The
below table shows a quick summary of what these changes mean for your site:
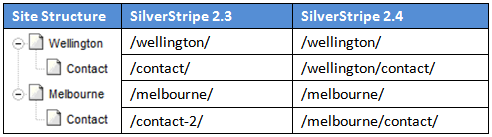
2011-02-07 07:48:44 +01:00
## Querying
Use *SiteTree::get_by_link()* to correctly retrieve a page by URL, as it taked nested URLs into account (a page URL
might consist of more than one *URLSegment* ).
:::php
// wrong
2012-06-23 00:32:43 +02:00
$mypage = SiteTree::get()->filter("URLSegment", '< mylink > ')->First();
2011-02-07 07:48:44 +01:00
// right
$mypage = SiteTree::get_by_link('< mylink > ');
2012-06-27 16:09:21 +02:00
### Versioning
The `SiteTree` class automatically has an extension applied to it: `[Versioned](api:Versioned)` .
This provides the basis for the CMS to operate on different stages,
and allow authors to save their changes without publishing them to
website visitors straight away.
`Versioned` is a generic extension which can be applied to any `DataObject` ,
so most of its functionality is explained in the `["versioning" topic](/topics/versioning)` .
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
Since `SiteTree` makes heavy use of the extension, it adds some additional
functionality and helpers on top of it.
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
Permission control:
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
:::php
class MyPage extends Page {
2012-09-19 12:07:39 +02:00
public function canPublish($member = null) {
2012-06-27 16:09:21 +02:00
// return boolean from custom logic
}
2012-09-19 12:07:39 +02:00
public function canDeleteFromLive($member = null) {
2012-06-27 16:09:21 +02:00
// return boolean from custom logic
}
}
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
Stage operations:
* `$page->doUnpublish()` : removes the "Live" record, with additional permission checks,
as well as special logic for VirtualPage and RedirectorPage associations
* `$page->doPublish()` : Inverse of doUnpublish()
* `$page->doRevertToLive()` : Reverts current record to live state (makes sense to save to "draft" stage afterwards)
* `$page->doRestoreToStage()` : Restore the content in the active copy of this SiteTree page to the stage site.
Hierarchy operations (defined on `[api:Hierarchy]` :
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
* `$page->liveChildren()` : Return results only from live table
* `$page->stageChildren()` : Return results from the stage table
* `$page->AllHistoricalChildren()` : Return all the children this page had, including pages that were deleted from both stage & live.
* `$page->AllChildrenIncludingDeleted()` : Return all children, including those that have been deleted but are still in live.
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
## Limiting Hierarchy
By default, any page type can be the child of any other page type.
However, there are static properties that can be
2011-02-07 07:48:44 +01:00
used to set up restrictions that will preserve the integrity of the page hierarchy.
2012-06-27 16:09:21 +02:00
Example: Restrict blog entry pages to nesting underneath their blog holder
2011-02-07 07:48:44 +01:00
:::php
class BlogHolder extends Page {
// Blog holders can only contain blog entries
2013-03-21 19:48:54 +01:00
private static $allowed_children = array("BlogEntry");
private static $default_child = "BlogEntry";
2012-06-27 16:09:21 +02:00
// ...
}
2011-02-07 07:48:44 +01:00
class BlogEntry extends Page {
// Blog entries can't contain children
2013-03-21 19:48:54 +01:00
private static $allowed_children = "none";
private static $can_be_root = false;
2012-06-27 16:09:21 +02:00
// ...
}
2011-02-07 07:48:44 +01:00
class Page extends SiteTree {
// Don't let BlogEntry pages be underneath Pages. Only underneath Blog holders.
2013-03-21 19:48:54 +01:00
private static $allowed_children = array("*Page,", "BlogHolder");
2011-02-07 07:48:44 +01:00
}
* **allowed_children:** This can be an array of allowed child classes, or the string "none" - indicating that this page
type can't have children. If a classname is prefixed by "*", such as "*Page", then only that class is allowed - no
subclasses. Otherwise, the class and all its subclasses are allowed.
* **default_child:** If a page is allowed more than 1 type of child, you can set a default. This is the value that
will be automatically selected in the page type dropdown when you create a page in the CMS.
* **can_be_root:** This is a boolean variable. It lets you specify whether the given page type can be in the top
level.
2012-06-27 16:09:21 +02:00
Note that there is no allowed_parents` control. To set this, you will need to specify the `allowed_children` of all other page types to exclude the page type in question.
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
## Permission Control
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
## Tree Display (Description, Icons and Badges)
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
The page tree in the CMS is a central element to manage page hierarchies,
hence its display of pages can be customized as well.
2011-02-07 07:48:44 +01:00
2012-06-27 16:09:21 +02:00
On a most basic level, you can specify a custom page icon
to make it easier for CMS authors to identify pages of this type,
when navigating the tree or adding a new page:
2011-02-07 07:48:44 +01:00
:::php
2012-06-27 16:09:21 +02:00
class StaggPage extends Page {
2013-03-21 19:48:54 +01:00
private static $singular_name = 'Staff Directory';
private static $plural_name = 'Staff Directories';
private static $description = 'Two-column layout with a list of staff members';
private static $icon = 'mysite/images/staff-icon.png';
2012-06-27 16:09:21 +02:00
// ...
2011-02-07 07:48:44 +01:00
}
2012-06-27 16:09:21 +02:00
You can also add custom "badges" to each page in the tree,
which denote status. Built-in examples are "Draft" and "Deleted" flags.
This is detailed in the ["Customize the CMS Tree" howto ](/howto/customize-cms-tree ).